An Introduction to the Riot API
A slightly different one for you guys this week — instead of showing you a statistical analysis, I’ll be teaching you how to do statistical analysis! We’re starting this series off with an introduction to the Riot API. You’ll find everything you need here to create your very first account analyser.
Before we start…
Do I need to know how to code?
No! Of course, it helps. But please, don’t let this put you off. When I first looked at the Riot API I didn’t know anything about coding and now I do it as a full time profession. You can learn the basics of coding AND the basics of the API at the same time. I won’t write a guide on learning Python since there’s millions out there, but hopefully the guide will give you the basics and you can go off and learn the rest yourself.
If you are new to Python, I recommend using Jupyter Notebooks — since it makes understanding what the code is doing a lot easier. You can download it here:
Install Jupyter Notebook through Anaconda.
I have also created this guide in the form of a notebook, which can be found here.
A whole bunch of people with various background knowledge have helped me test this guide out, if you need some help you can join the iTero Discord and get advise, fix issues or find out more in the #riot-api-learners channel.
Getting Setup on the Riot API Developer Portal
To begin working with the Riot API, we must first set-up an account on the portal. Head over to: https://developer.riotgames.com/ and sign-in using your Riot account.
Once you’re in, head down to “REGENERATE YOUR API KEY” to get a temporary pass (lasts 24 hours). Remember, never share this key with anyone else and be careful where you store it! You can then copy it over to your Python interpreter, if you’re following the guide exactly it’ll be Jupyter Notebook.
Our First Riot API Call
It’s time to make our first call to the Riot API.
In order to do this, we need to find the API that we want to call, which is in the form of a URL. To find all the ones available to you, tab back into the developer portal and press “APIs”. On your left should be a list of all the available types of calls to be made — don’t be overwhelmed, they cover all Riot games and in reality you’ll only need to use a small number of them.
Scroll down until you see “SUMMONER-V4”, once you click it a list of URLs will appear. You’re looking for the one called
/lol/summoner/v4/summoners/by-name/{summonerName}.
Go down the page until you reach the “PATH PARAMETERS”. Enter your desired Summoner Name, the region that Summoner is in and then ensure the “SELECT APP TO EXECUTE AGAINST” is set to “Development API Key”. Then hit EXECUTE REQUEST.
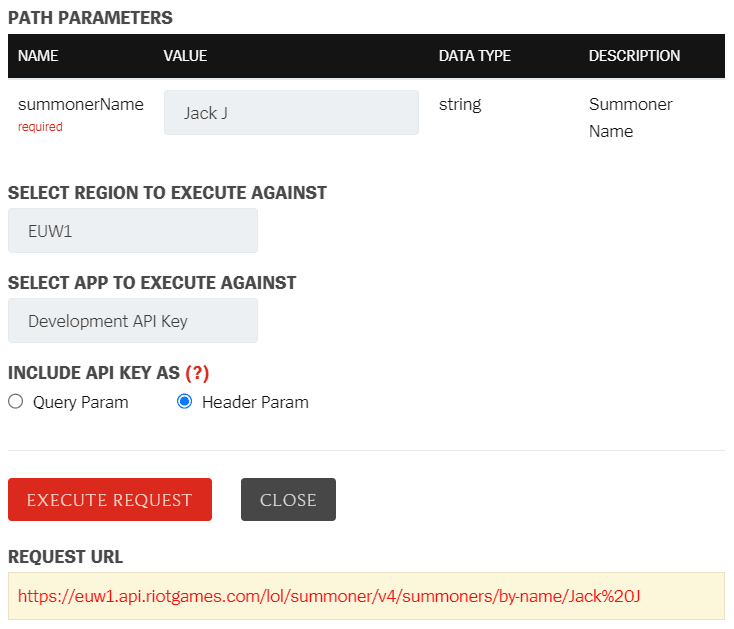
If everything’s gone well, you should get a “RESPONSE CODE” of 200. Copy the “REQUEST URL” into Python.
It should look something like this:
api_key = "YOUR_KEY"
api_url = "https://euw1.api.riotgames.com/lol/summoner/v4/summoners/by-name/Jack%20J"
To manage our API requests we’ll be using a Python package called “requests”, which is usually preinstalled (if you get an error saying “No module named ‘requests’”, you’ll need to do some Googling on how to install packages).
Sending a request is easy:
import requests requests.get(api_url)
However, you’ll get an error code 401. You can check on the developer portal to find out what each code means. This one refers to “Unauthorized”. This is because we haven’t attached our API key to the request, and so the internet bouncers won’t let you in to get the data.
When dealing with APIs, we can tag on arguments at the end of the URL by adding “?” at the end. Like this:
api_url = api_url + '?api_key=' + api_key
Which makes the URL:
https://euw1.api.riotgames.com/lol/summoner/v4/summoners/by-name/Jack%20J?api_key=YOUR_API_KEY
Now, go back and resend the URL. This time you should receive the desired “200” response, which indicate it’s been successful. But where’s the data? Here’s how we extract it from the API response:
resp = requests.get(api_url)
player_info = resp.json()
The “player_info” object should look something like this:
{
'id': 'gkjv-w3_gkWbFFmMZkrDj2JR-rrmv4Mj172gqRO6ycmY9FY',
'accountId': 'aD6DoTQb_RWYLAghp_mWwuwAWHOW6qRz6zgQsf2y5YIitQ',
'puuid': 'dIWQhEOVCY9QT93Jz0EX6YxQIfI3zsyL7tVNmyApKpPmvgNnq5zBu-VKtgGvt2bWzj-fQqd1mYVZMA',
'name': 'Jack J',
'profileIconId': 918,
'revisionDate': 1658870637000,
'summonerLevel': 346
}
Getting Match Data
Sending our first API call was fairly straight-forward, except it doesn’t give us much information to work with. We’ll now look at getting historic match data for our account.
To do this, we must first work out what the API call needs to be. Again, go back to the portal and this time find “MATCH-V5” on the far left. Go to the option called: /lol/match/v5/matches/by-puuid/{puuid}/ids
. The {puuid}
indicates that we need to insert a puuid into the URL in order to use it. Luckily we got one from our first API call (go back up and check the “player_info” to find the puuid).
We can then copy and paste the puuid into the “PATH PARAMETERS” on the developer portal. Ensure the region and API key are also request, leave the remaining fields as they are and then EXECUTE REQUEST.
Once again, copy the REQUEST URL back into Python, it’ll look something like this:
api_url = "https://europe.api.riotgames.com/lol/match/v5/matches/by-puuid/dIWQhEOVCY9QT93Jz0EX6YxQIfI3zsyL7tVNmyApKpPmvgNnq5zBu-VKtgGvt2bWzj-fQqd1mYVZMA/ids?start=0&count=20"
Note, if you inspect the URL you’ll see there’s already two arguments at the end: “?start=0&count=20”. This means if we want to add a new argument (the API key), we’ll have to add “&” instead of “?”
Once you’ve done that, send the URL off the same as before:
api_url = api_url + '&api_key=' + api_key
resp = requests.get(api_url)
match_ids = resp.json()
The match_ids will look something like this:
[
'EUW1_5987369961',
'EUW1_5987334405',
'EUW1_5987297136',
'EUW1_5985953834',
'EUW1_5985877576',
'EUW1_5984051808',
'EUW1_5983630065',
'EUW1_5983595510',
'EUW1_5982943551',
'EUW1_5982849305',
'EUW1_5982852241',
'EUW1_5982834160',
'EUW1_5982385567',
'EUW1_5982421620',
'EUW1_5981636284',
'EUW1_5981413357',
'EUW1_5981269044',
'EUW1_5981244521',
'EUW1_5980174276',
'EUW1_5980007970'
]
This contains a list of match IDs for the accounts most recent 20 games.
In order to find out more information about one of these matches we can use another API, which you can find in the portal called /lol/match/v5/matches/{matchId}
.
In the portal, scroll down and copy one of the match IDs into the “matchId” input, ensuring the region and API key are the same as before. We can then copy the API URL and send it off through Python.
api_url = "https://europe.api.riotgames.com/lol/match/v5/matches/EUW1_5982385567"
This one doesn't have any arguments (see, there's no "?" above) so we'll add it with the api key
api_url = api_url + '?api_key=' + api_key
resp = requests.get(api_url)
match_data = resp.json()
If all goes well you’ll find A LOT of fields in the “match_data” object. It contains all the information about the match such as game length, start time and which puuids were in the game. It also contains information about each player and their performance, such as KDA or their champions name. Let’s take a look at one of the players KDA:
To save time, we'll assign a variable for the first player
player_data = match_data['info']['participants'][0]k = player_data['kills']
d = player_data['deaths']
a = player_data['assists']
print("Kills:", k)
print("Deaths:", d)
print("Assists:", a)
print("KDA:", (k + a) / d)
However, we don’t want the information about any random player in that game! We want to find out how the summoner we searched for originally had performed. In order to do this, we need to find in which order they appear.
To do this, grab a list of all the participant puuids and use the “index” function to find out where they are.
A list of all the participants puuids
participants = match_data['metadata']['participants']
Now, find where in the data our players puuid is found
player_index = participants.index(puuid)
Hopefully the name below is what you inputted into the first function!
print(match_data['info']['participants'][player_index]['summonerName'])
Once we’ve found where they are, we can print out some information specifically about them:
player_data = match_data['info']['participants'][player_index]champ = player_data['championName']
k = player_data['kills']
d = player_data['deaths']
a = player_data['assists']
win = player_data['win']print("Champ:", champ, "Kills:", k, "Deaths:", d, "Assists:", a, "Win:", win)
That's as far as we'll take it here, since the rest is heavier on the code side and it's much easier to just see it in action in the notebook. Hopefully that gives you a reasonable understanding on making your first Riot API call. Join the Discord if you need any help!